Connectez-vous pour activer le suivi
Abonnés
0
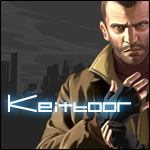
Tutorial #3 - Crazy Clothes
Par
Keitboor, dans GTA San Andreas
-
En ligne récemment 0 membre est en ligne
Aucun utilisateur enregistré regarde cette page.